let's get IT with DAVINA ๐ป
React-Styled Components ๋ณธ๋ฌธ
Styled Components
- ์ปดํฌ๋ํธ๋ฅผ ๊ธฐ๋ฐ์ผ๋ก css๋ฅผ ์์ฑํ ์ ์๊ฒ ๋์์ฃผ๋ ๋ผ์ด๋ธ๋ฌ๋ฆฌ
- ์ฐ๋ฆฌ๊ฐ ์๊ณ ์๋ css๋ฅผ ๊ทธ๋๋ก ์ฌ์ฉํ ์ ์๋ค.
- css๋ฅผ ์ปดํฌ๋ํธ ์์ผ๋ก ์บก์ํํ์ฌ ๋ค์ด๋ฐ์ด๋ ์ต์ ํ๋ฅผ ์ ๊ฒฝ ์ธ ํ์๊ฐ ์์
- ๋น๊ต์ ๋น ๋ฅธ ํ์ด์ง ๋ก๋์ ๋ถ๋ฆฌ
Styled Components ์ค์น๋ฒ
- ํฐ๋ฏธ๋์์ Styled Components ๋ผ์ด๋ธ๋ฌ๋ฆฌ ์ค์น
# with npm
$ npm install --save styled-components
# with yarn
$ yarn add styled-components
- package.json์ ์๊ธฐ ์ฝ๋ ์ถ๊ฐ ๊ถ์ฅ (์ฌ๋ฌ ๋ฒ์ ์ค์น ๋ฐฉ์ง)
{
"resolutions": {
"styled-components": "^5"
}
}
- ํ์ํ ๋๋ง๋ค importํด์ ์ฌ์ฉ
import styled from "styled-components"
Styled Components ์ฌ์ฉ๋ฒ
1. ์ ์ญ ์คํ์ผ ์ง์ ํ๊ธฐ
์ ์ญ์คํ์ผ์ ๋ชจ๋ html ์์์ ๋ํด์ ๊ณตํต์ ์ธ ์คํ์ผ์ ๊ฐ์ง๋ค.
ex)
- ๋ชจ๋ li ํ๊ทธ์์ list-style ์ ๊ฑฐํ๊ธฐ
- a ํ๊ทธ์์ text-decoration ์ ๊ฑฐํ๊ธฐ
- button ํ๊ทธ์ cursor: pointer ์ง์ ํ๊ธฐ
reset CSS๋ฅผ ํตํด ์ด๊ธฐํํ๊ธฐ ๐ฝ
- src/style/GlobalStyle.jsx
import { createGlobalStyle } from "styled-components";
import reset from "styled-reset";
const GlobalStyle = createGlobalStyle`
${reset}
:root {
*{
box-sizing: border-box;
outline: none;
list-style: none;
text-decoration: none;
font-family: 'Noto Sans KR', sans-serif;
::-webkit-scrollbar {
display: none;
}
user-select: none;
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
}
}
`;
export default GlobalStyle;
theme ์ง์ (๋ด๊ฐ ์ธ ์์๋ค ํ๋ ํธ ๋ง๋ค๊ธฐ)
- src/style/Theme.jsx
export const colorTheme = {
darkBlack: "#000",
mediumBlack: "#333",
lightBlack: "#4a4a4a",
darkGrey: "#999",
mediumGrey: "#a4a4a4",
lightGrey: "#ccc",
white: "#fff",
pointColor: "#20b2aa",
};
2. ์ปดํฌ๋ํธ ๋ง๋ค๊ธฐ
Styled Components๋ ES6์ Templete Literals ๋ฌธ๋ฒ์ ์ฌ์ฉํฉ๋๋ค. ์ฆ, ๋ฐ์ดํ๊ฐ ์๋ ๋ฐฑํฑ(`)์ ์ฌ์ฉํฉ๋๋ค.
const ์ปดํฌ๋ํธ์ด๋ฆ = styled.ํ๊ทธ์ข
๋ฅ`
css์์ฑ1: ์์ฑ๊ฐ;
css์์ฑ2: ์์ฑ๊ฐ;
`
import styled from "styled-components";
//Styled Components๋ก ์ปดํฌ๋ํธ๋ฅผ ๋ง๋ค๊ณ
const BlueButton = styled.button`
background-color: blue;
color: white;
`;
export default function App() {
// React ์ปดํฌ๋ํธ๋ฅผ ์ฌ์ฉํ๋ฏ์ด ์ฌ์ฉํ๋ฉด ๋ฉ๋๋ค.
return <BlueButton>Blue Button</BlueButton>;
}
3. ์ปดํฌ๋ํธ๋ฅผ ์ฌํ์ฉํด์ ์๋ก์ด ์ปดํฌ๋ํธ ๋ง๋ค๊ธฐ
const ์ปดํฌ๋ํธ์ด๋ฆ = styled(์ฌํ์ฉํ ์ปดํฌ๋ํธ)`
์ถ๊ฐํ css์์ฑ1: ์์ฑ๊ฐ;
์ถ๊ฐํ css์์ฑ2: ์์ฑ๊ฐ;
`
//๋ง๋ค์ด์ง ์ปดํฌ๋ํธ๋ฅผ ์ฌํ์ฉํด ์ปดํฌ๋ํธ๋ฅผ ๋ง๋ค ์ ์์ต๋๋ค.
const BigBlueButton = styled(BlueButton)`
padding: 10px;
margin-top: 10px;
`;
//์ฌํ์ฉํ ์ปดํฌ๋ํธ๋ฅผ ์ฌํ์ฉํ ์๋ ์์ต๋๋ค.
const BigRedButton = styled(BigBlueButton)`
background-color: red;
`;
export default function App() {
return (
<>
<BlueButton>Blue Button</BlueButton>
<br />
<BigBlueButton>Big Blue Button</BigBlueButton>
<br />
<BigRedButton>Big Red Button</BigRedButton>
</>
);
}
4. Props ํ์ฉํ๊ธฐ
Styled Components๋ ํ ํ๋ฆฟ ๋ฆฌํฐ๋ด ๋ฌธ๋ฒ( ${ } )์ ์ฌ์ฉํ์ฌ JavaScript ์ฝ๋๋ฅผ ์ฌ์ฉํ ์ ์์ต๋๋ค. props๋ฅผ ๋ฐ์์ค๋ ค๋ฉด props๋ฅผ ์ธ์๋ก ๋ฐ๋ ํจ์๋ฅผ ๋ง๋ค์ด ์ฌ์ฉํ๋ฉด ๋ฉ๋๋ค.
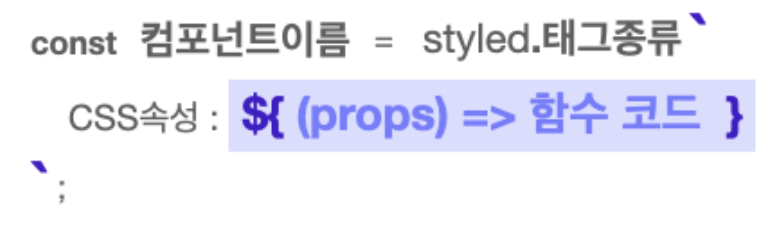
- Props๋ก ์กฐ๊ฑด๋ถ ๋ ๋๋งํ๊ธฐ

import styled from "styled-components";
import GlobalStyle from "./GlobalStyle";
//๋ฐ์์จ prop์ ๋ฐ๋ผ ์กฐ๊ฑด๋ถ ๋ ๋๋ง์ด ๊ฐ๋ฅํฉ๋๋ค.
const Button1 = styled.button`
background: ${(props) => (props.skyblue ? "skyblue" : "white")};
`;
export default function App() {
return (
<>
<GlobalStyle />
<Button1>Button1</Button1>
<Button1 skyblue>Button1</Button1>
</>
);
}
Props ๊ฐ์ผ๋ก ๋ ๋๋งํ๊ธฐ
- props์ ๊ฐ์ ํต์งธ๋ก ํ์ฉํด์ ์ปดํฌ๋ํธ ๋ ๋๋ง์ ํ์ฉํ ์ ์์ต๋๋ค.
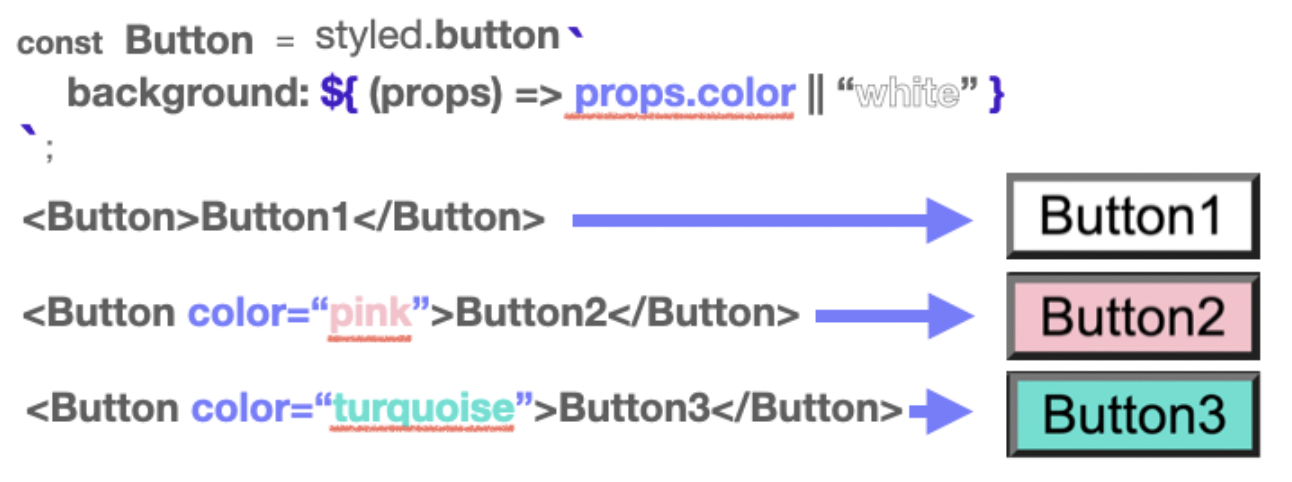
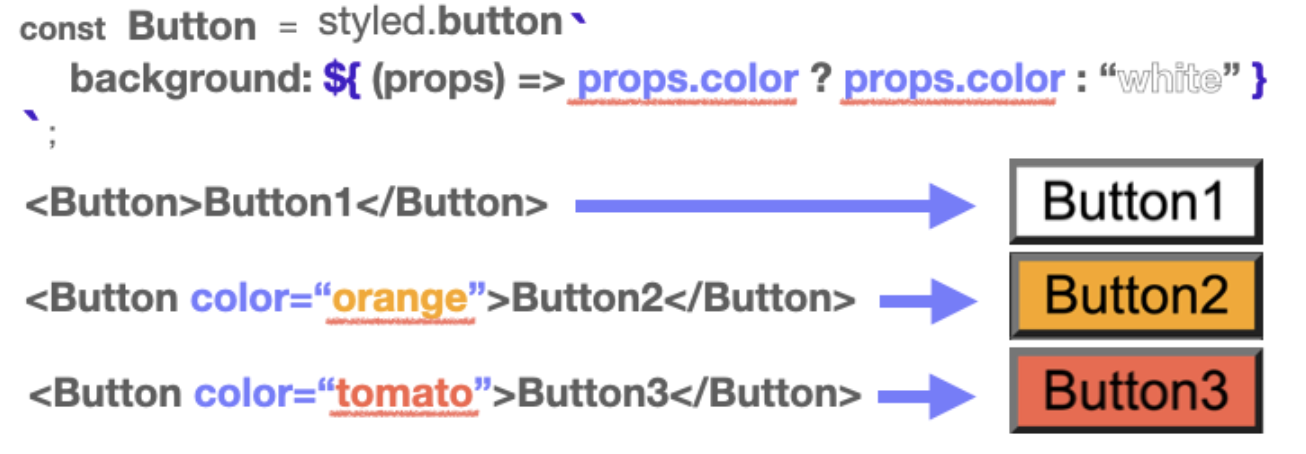
5. ThemeProvider ์ฌ์ฉํ๊ธฐ
- styled-components์์ ThemeProvider๋ฅผ importํ๋ค.
import { ThemeProvider } from "styled-components";
- ThemeProvider ์ปดํฌ๋ํธ๋ฅผ ๊ฐ์ฅ ์์ ์ปดํฌ๋ํธ๋ก ๋ฐฐ์นํ๋ค. (GlobalStyle๋ณด๋ค ์์)
function App() {
return (
<ThemeProvider>
<GlobalStyle />
<BrowserRouter>
<Switch>
<Route path="/login">
<LogIn />
</Route>
<Route path="/">
<Home />
</Route>
</Switch>
</BrowserRouter>
</ThemeProvider>
);
}
- theme์ด๋ผ๋ ์ด๋ฆ์ ๊ฐ์ง ๋น์ด์๋ ๊ฐ์ฒด๋ฅผ ๋ง๋ ๋ค.
const theme = {}
- ์์ฑํ theme ๊ฐ์ฒด๋ฅผ ThemeProvider์ props๋ก ๋ฃ์ด์ค๋ค.
- ๊ทธ๋ฌ๋ฉด styled-components๊ฐ ๋ชจ๋ ์ปดํฌ๋ํธ์ ์ด theme ๋ณ์๋ฅผ injectํด์ค๋ค.
function App() {
return (
<ThemeProvider theme={theme}>
<GlobalStyle />
<BrowserRouter>
<Switch>
<Route path="/login">
<LogIn />
</Route>
<Route path="/">
<Home />
</Route>
</Switch>
</BrowserRouter>
</ThemeProvider>
);
}
- ์ํ๋ theme ์์ฑํ๊ธฐ (camelCase)
const theme = {
primaryColor: "#f8049c",
secondaryColor: " #fdd54f",
};
์ ์ฉ์ ๐ฝ
${props => props.theme.primaryColor}
//ex)
background-color: ${(props)=>
props.secondary ? props.theme.secondaryColor : props.themeprimaryColor};
border-bottom: 3px solid ${(props) => props.theme.secondaryColor};
Styled Component ์ด๋ฒคํธ ์ฃผ๊ธฐ
- App.js
import styled from "styled-components"; //์คํ์ผ๋์ปดํฌ๋ํธ ์ ์ฉ
import GlobalStyle from "./GlobalStyle"; //์ ์ญ์คํ์ผ ์ ์ฉ
const BlueButton = styled.button`
background-color: powderblue;
padding: 1rem;
font-size: 2rem;
border-radius: 1rem;
transition: 0.5s;
//hover ์ด๋ฒคํธ ์ฃผ๊ธฐ!!!
&:hover {
background: cornflowerblue;
color: white;
transition: 0.5s;
}
`;
export default function App() {
return (
<>
<GlobalStyle />
<BlueButton>Practice!</BlueButton>
</>
);
}
- GlobalStyle.js โ ์ ์ญ์คํ์ผ ์ค์ ํ๊ธฐ
import { createGlobalStyle } from "styled-components";
const GlobalStyle = createGlobalStyle`
* { margin: 0.5rem; }
`;
export default GlobalStyle;
'DEV_IN > CSS' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
div์ img ๋ฃ๊ธฐ (props) (0) | 2023.02.09 |
---|---|
๊ธ์ ์ ์ด๊ณผ์? (0) | 2023.02.09 |
Position (0) | 2023.02.09 |
GMT/UTC time ํ์ โ2023-02-02โ format์ผ๋ก change (0) | 2023.02.08 |
react-datepicker (2) | 2023.02.08 |
Comments